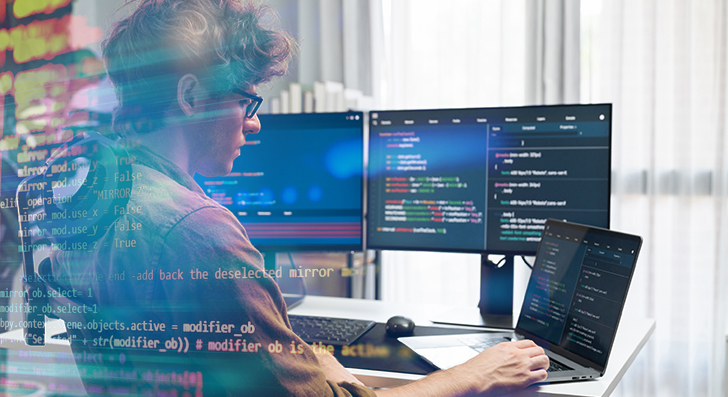
Scalability means your application can deal with growth—extra end users, more facts, plus much more targeted traffic—with out breaking. As a developer, making with scalability in mind will save time and anxiety later on. Here’s a transparent and sensible guideline to assist you to commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on later on—it should be aspect of one's system from the beginning. Quite a few applications fall short once they grow rapidly because the initial design can’t take care of the additional load. As being a developer, you'll want to Believe early regarding how your method will behave stressed.
Start by developing your architecture to generally be flexible. Keep away from monolithic codebases where every little thing is tightly related. As an alternative, use modular structure or microservices. These patterns split your application into lesser, independent elements. Just about every module or service can scale on its own devoid of affecting the whole program.
Also, contemplate your databases from working day 1. Will it want to manage one million customers or maybe 100? Pick the ideal type—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t want them nevertheless.
A different significant issue is to stay away from hardcoding assumptions. Don’t write code that only functions below present situations. Contemplate what would materialize In the event your person foundation doubled tomorrow. Would your application crash? Would the database decelerate?
Use design styles that aid scaling, like message queues or occasion-driven systems. These help your application tackle extra requests without the need of finding overloaded.
Any time you Make with scalability in your mind, you are not just getting ready for success—you're reducing upcoming complications. A properly-planned method is easier to maintain, adapt, and mature. It’s superior to organize early than to rebuild later.
Use the Right Databases
Selecting the correct databases can be a critical Section of developing scalable purposes. Not all databases are designed precisely the same, and using the Completely wrong one can slow you down or maybe bring about failures as your app grows.
Get started by knowledge your info. Can it be hugely structured, like rows inside a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient suit. These are solid with relationships, transactions, and regularity. They also assist scaling methods like examine replicas, indexing, and partitioning to deal with additional site visitors and information.
If the information is a lot more flexible—like consumer exercise logs, solution catalogs, or files—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at managing big volumes of unstructured or semi-structured facts and can scale horizontally a lot more conveniently.
Also, think about your examine and write designs. Will you be doing a lot of reads with fewer writes? Use caching and read replicas. Will you be managing a heavy compose load? Check into databases that can manage substantial generate throughput, or even celebration-centered data storage techniques like Apache Kafka (for short term facts streams).
It’s also good to think ahead. You may not want Innovative scaling capabilities now, but choosing a database that supports them implies you gained’t need to have to change later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your facts based upon your obtain patterns. And usually check database efficiency as you develop.
In brief, the correct database depends upon your app’s structure, speed needs, and how you expect it to mature. Choose time to select correctly—it’ll preserve plenty of problems later.
Improve Code and Queries
Quick code is essential to scalability. As your application grows, every single modest delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s imperative that you Make economical logic from the beginning.
Commence by writing clean up, uncomplicated code. Stay away from repeating logic and remove just about anything unwanted. Don’t choose the most complex Option if a straightforward a single works. Keep the functions shorter, centered, and easy to check. Use profiling resources to uncover bottlenecks—spots exactly where your code usually takes far too extended to operate or employs an excessive amount of memory.
Upcoming, examine your databases queries. These usually gradual items down much more than the code by itself. Be certain Just about every query only asks for the information you truly want. Stay clear of Pick *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Specially throughout big tables.
When you notice the identical facts becoming requested time and again, use caching. Store the outcomes briefly applying tools like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your databases functions when you can. As opposed to updating a row one by one, update them in teams. This cuts down on overhead and would make your application more effective.
Remember to exam with large datasets. Code and queries that function fantastic with one hundred data could crash every time they have to handle 1 million.
In short, scalable apps are quick apps. Keep your code restricted, your queries lean, and use caching when wanted. These techniques assistance your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to manage additional people plus more targeted visitors. If every thing goes by means of a single server, it's going to rapidly become a bottleneck. That’s where load balancing and caching come in. Both of these instruments enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming targeted visitors across various servers. In lieu of just one server executing every one of the perform, the load balancer routes customers to different servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other folks. Resources like Nginx, HAProxy, or cloud-based alternatives from AWS and Google Cloud make this simple to setup.
Caching is about storing details briefly so it can be reused immediately. When end users request a similar facts once more—like an item website page or even a profile—you don’t need to fetch it with the database when. It is possible to serve it with the cache.
There are two prevalent varieties of caching:
one. Server-side caching (like Redis or Memcached) suppliers info in memory for speedy accessibility.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t improve normally. And often be certain your cache is up to date when facts does change.
In a nutshell, load balancing and caching are very simple but effective instruments. Together, they help your application handle a lot more people, stay quickly, and recover from difficulties. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you may need applications that let your app develop simply. That’s wherever cloud platforms and containers are available. They give you flexibility, lower set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy hardware or guess future capacity. When visitors raises, you'll be able to incorporate extra means with just some clicks or quickly applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also give read more products and services like managed databases, storage, load balancing, and stability instruments. It is possible to target constructing your app rather than managing infrastructure.
Containers are another important tool. A container offers your application and almost everything it has to run—code, libraries, configurations—into just one unit. This makes it quick to maneuver your app between environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Software for this.
Whenever your app takes advantage of many containers, resources like Kubernetes help you regulate them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the app crashes, it restarts it mechanically.
Containers also allow it to be straightforward to individual parts of your application into solutions. You could update or scale areas independently, which can be perfect for functionality and reliability.
Briefly, utilizing cloud and container instruments indicates you could scale quickly, deploy conveniently, and Recuperate immediately when difficulties materialize. If you'd like your application to grow with no limits, commence applying these instruments early. They save time, minimize hazard, and assist you to keep centered on developing, not repairing.
Watch Every thing
In case you don’t observe your application, you won’t know when factors go Completely wrong. Monitoring aids the thing is how your application is accomplishing, spot concerns early, and make improved decisions as your app grows. It’s a essential Component of building scalable methods.
Commence by monitoring primary metrics like CPU use, memory, disk House, and response time. These tell you how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you obtain and visualize this data.
Don’t just keep track of your servers—keep track of your app also. Keep watch over how much time it's going to take for buyers to load internet pages, how frequently faults materialize, and where by they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s going on within your code.
Build alerts for vital complications. Such as, In the event your reaction time goes previously mentioned a limit or even a services goes down, you need to get notified instantly. This helps you fix problems fast, often right before people even observe.
Monitoring is also practical any time you make alterations. Should you deploy a brand new feature and find out a spike in problems or slowdowns, you are able to roll it again ahead of it triggers real destruction.
As your app grows, visitors and details enhance. With out checking, you’ll miss out on signs of trouble until eventually it’s also late. But with the right instruments in place, you keep in control.
Briefly, monitoring can help you keep your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about being familiar with your program and making sure it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for big corporations. Even small applications require a robust foundation. By planning carefully, optimizing properly, and utilizing the correct instruments, you can Create applications that develop efficiently without breaking under pressure. Start out small, Feel major, and build sensible.